top of page
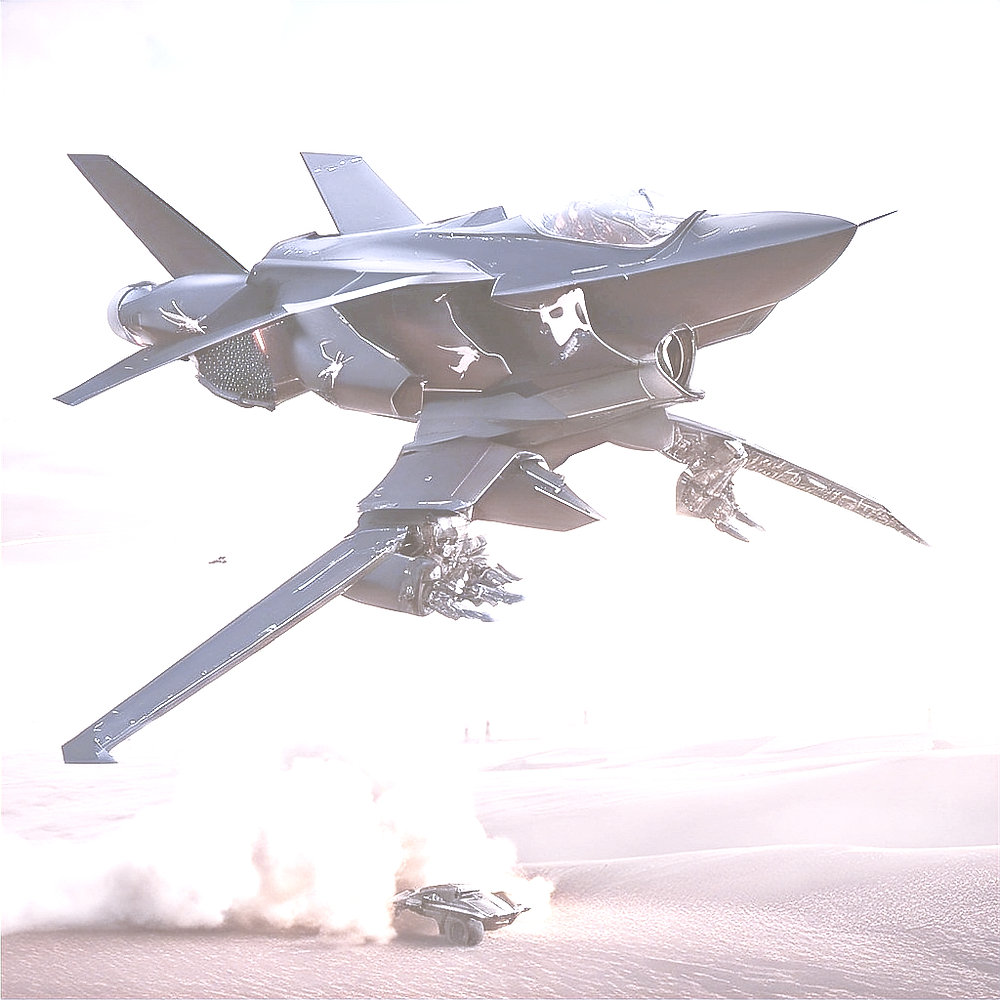
Display images on screen in grid view & video as header with Responsive Web design code
Sep 13, 2024
2 min read
0
11
2
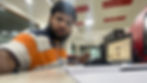
Suppose i have 12 images and i want to display them in such a way that for desktop view I get 3 images per row and for mobile version , i get 2 images per row , then a fixed code that you can use is below :
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Grid with Video Header</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<div class="video-container">
<video autoplay muted loop class="background-video">
<source src="videoplayback.mp4" type="video/mp4">
Your browser does not support the video tag.
</video>
</div>
<div class="header-content">
<h1>Welcome to My Website</h1>
</div>
</header>
<div class="image-grid">
<div class="image-item">
<img src="image1.png" alt="Image 1">
<p>Image 1</p>
</div>
<div class="image-item">
<img src="image2.png" alt="Image 2">
<p>Image 2</p>
</div>
<div class="image-item">
<img src="image3.png" alt="Image 3">
<p>Image 3</p>
</div>
<div class="image-item">
<img src="image4.png" alt="Image 4">
<p>Image 4</p>
</div>
<div class="image-item">
<img src="image5.png" alt="Image 5">
<p>Image 5</p>
</div>
<div class="image-item">
<img src="image6.jpg" alt="Image 6">
<p>Image 6</p>
</div>
<div class="image-item">
<img src="image7.png" alt="Image 7">
<p>Image 7</p>
</div>
<div class="image-item">
<img src="image8.png" alt="Image 8">
<p>Image 8</p>
</div>
<div class="image-item">
<img src="image9.png" alt="Image 9">
<p>Image 9</p>
</div>
<div class="image-item">
<img src="image10.png" alt="Image 10">
<p>Image 10</p>
</div>
<div class="image-item">
<img src="image11.png" alt="Image 11">
<p>Image 11</p>
</div>
<div class="image-item">
<img src="image12.png" alt="Image 12">
<p>Image 12</p>
</div>
</div>
</body>
</html>
CSS with media quries:
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
box-sizing: border-box; /* Ensure padding and border are included in width and height */
}
header {
position: relative;
width: 100%;
height: 50vh; /* Adjust height as needed */
border: 5px solid blue; /* Blue border around header */
box-sizing: border-box; /* Include border in the header's size */
}
.video-container {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
.background-video {
width: 100%;
height: 100%;
object-fit: cover; /* Ensures the video covers the container while maintaining aspect ratio */
}
.header-content {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
color: white;
text-align: center;
z-index: 1; /* Ensures content appears above the video */
}
.header-content h1 {
margin: 0;
font-size: 2rem; /* Adjust font size as needed */
}
/* Grid layout for images */
.image-grid {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
gap: 10px;
padding: 10px;
}
.image-item {
text-align: center;
}
.image-item img {
width: 100%;
height: auto;
border-radius: 12px; /* Slightly rounded corners */
border: 2px solid black; /* Black border */
}
.image-item p {
margin: 5px 0 0;
}
/* Media query for desktop devices */
@media (min-width: 768px) {
.image-grid {
grid-template-columns: repeat(3, 1fr);
}
}
/* Media query for mobile devices */
@media (max-width: 767px) {
.image-grid {
grid-template-columns: repeat(2, 1fr);
}
}
Images have round corner black border and header as video with blue corder ,in responsive design format .
https://udditwork.github.io/grid-view-images-with-videoheader/

Related Posts
Comments (2)
bottom of page
lodu he tu